Ruby on Rails Interview Questions with Answers
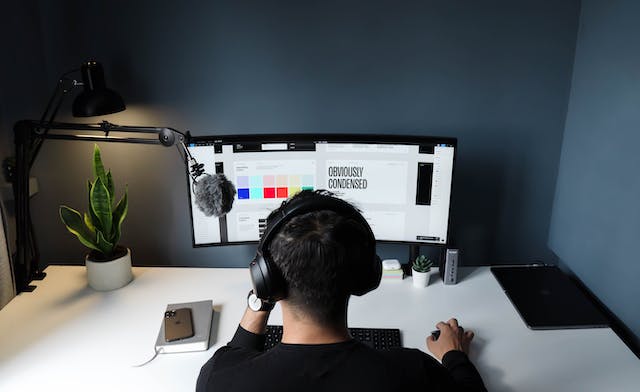
Ruby on Rails remains a crucial tool in web development, known for its efficiency and flexibility. For companies looking to hire top talent, understanding Ruby on Rails interview questions is key. That’s why we’ve created a comprehensive list of questions to help you find the right candidate. These questions cover both basic and advanced skills, ensuring a thorough assessment. With the right questions, you’ll discover the perfect match for your team. Let us help you streamline your hiring process today!
What is Ruby on Rails?
Ruby on Rails, or Rails, is a web framework in Ruby. It prioritizes convention over configuration and the DRY principle to streamline web development.
Explain the MVC architecture as it pertains to Rails.
Rails follows the Model-View-Controller (MVC) architecture. The Model manages the database, the View handles the user interface, and the Controller processes events, interacting with model objects.
What are migrations in Rails?
Migrations in Rails are a way to alter the database schema over time in a consistent and easy way. They use a Ruby DSL so that you don’t have to write SQL by hand, allowing for database-agnostic code.
Describe the asset pipeline in Rails.
The asset pipeline provides a framework to concatenate and minify or compress JavaScript and CSS assets. It also allows for coding assets via a higher-level language like Sass or CoffeeScript.
How do you create a new Rails project?
To create a new Rails project, use the command rails new project_name
, which sets up a new Rails directory with all the necessary installation files.
What is the use of the bundle exec
command?
Bundle exec runs a command within the context of the current project’s Gemfile, ensuring it uses the specified gems and versions.
Explain how you can define routes in Rails.
Routes in Rails are defined in the config/routes.rb
file. This configuration file tells Rails how to connect incoming requests to controllers and actions.
What is ActiveRecord and how does it work?
ActiveRecord is the M model in MVC, serving as an ORM (Object-Relational Mapping) framework for Rails. It represents data as objects without requiring long SQL scripts.
How are filters used in Rails?
Rails runs filters “before,” “after,” or “around” a controller action to handle tasks like authentication and authorization.
What is CSRF protection and how does Rails help enforce it?
Rails enforces CSRF protection to prevent attacks that transmit unauthorized commands from trusted users. It includes a security token in forms and ensures AJAX requests check the token.
What are Gems and how are they used?
Developers use gems as reusable libraries in Rails applications to add functionality. You can install them with the gem install command or by adding them to the Gemfile and running bundle install.
Explain the concept of “Convention over Configuration”.
“Convention over Configuration” means that Rails has defaults for most things, which reduces the number of decisions a developer needs to make without losing flexibility.
How does Rails handle database transactions?
Rails handles database transactions by wrapping the operations that are to be executed as a single atomic operation in a transaction
block. If any operation fails, the entire transaction is rolled back.
What is the flash, and how do developers use it?
Rails stores temporary messages in the flash object to display after performing an action, typically for errors or confirmations.
Describe polymorphic associations in Rails.
Polymorphic associations allow a model to belong to more than one other model, on a single association. For example, you might have a Picture model that belongs to either an Employee model or a Product model.
How do you manage multiple forms of authentication in Rails?
Rails manages multiple forms of authentication by using gems like Devise or Authlogic, which offer customizable solutions, or by manually implementing session-based authentication methods.
What are Rails engines?
Rails engines act as mini-applications, providing functionality to their host applications. Developers reuse them across different projects, as they include their own MVC components.
Explain how developers use callbacks in Rails.
Callbacks in Rails are hooks into the lifecycle of an Active Record object that allow you to trigger logic before or after an alteration of the object state. This includes creating, saving, updating, deleting.
How does caching work in Rails?
Rails improves performance by caching expensive output or calculations. Developers can apply caching at the page, action, or fragment level.
What is the purpose of the yield
keyword in Rails views?
The yield
keyword is used in Rails layouts to include the content from views into a common layout structure, like headers and footers, which surrounds different pages within an application.
How do you manage database-dependent settings in Rails?
Rails manages database-dependent settings by using environment variables and custom initializers to keep sensitive data out of the application code.
What is the difference between DateTime
, Timestamp
, Time
and Date
in Rails?
DateTime
, Timestamp
, Time
, and Date
are different classes in Rails used for handling dates and times. DateTime
and Timestamp
include date and time, Time
is only concerned with time, and Date
handles only date.
What are the different types of associations in Rails?
Rails supports several types of associations: belongs_to
, has_many
, has_one
, and has_and_belongs_to_many
, allowing models to interact with each other through database relationships.
How do you perform error handling in Rails?
Error handling in Rails can be performed using the rescue_from
method within controllers to manage different types of exceptions in a centralized way.
What are namespaces, and how do developers use them in Rails routing?
Rails uses namespaces in routing to group related actions under a common path prefix, often for administrative functions with a shared layout.
Explain the use of the respond_to
method in Rails.
The respond_to
method in Rails is used in controllers to respond differently depending on the format of the request, such as HTML, JSON, or XML, allowing the same URL to serve different types of data.
How do you optimize a Rails application?
Optimizing a Rails application can involve database index additions, query optimization, use of the asset pipeline for CSS and JS, background job processing for lengthy tasks, and caching strategies.
Common Technologies Used with Ruby on Rails
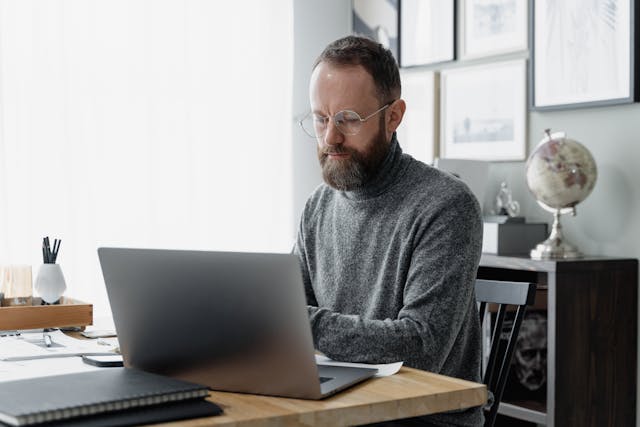
Ruby on Rails, as a comprehensive web application framework, leverages a variety of technologies to enhance its functionality and efficiency. Here are some of the most common technologies used alongside Rails:
- Ruby: The core programming language for Rails. It is dynamic, reflective, and object-oriented, making it ideal for the concise and readable code Rails is known for.
- JavaScript: Often used to add interactivity to Rails applications. Frameworks like React, Vue.js, or Angular can be integrated with Rails to enhance client-side functionality.
- HTML & CSS: Essential for designing the frontend of Rails applications, often managed within Rails through template engines like ERB (Embedded Ruby).
- SQL Database Systems: Rails typically uses databases such as PostgreSQL, MySQL, or SQLite to store application data, managed through ActiveRecord as part of Rails’ ORM system.
- Webpack: Integrated into Rails through the Webpacker gem, it helps manage JavaScript, CSS, and assets compilation.
- Redis: Frequently used for caching in Rails applications to enhance performance by storing session and cache data.
- Sidekiq: A background processing tool for Ruby that integrates seamlessly with Rails for handling background jobs like email notifications or batch imports.
- Elasticsearch: A search engine that can be integrated into Rails applications for powerful search capabilities, often used for complex query handling and data indexing.
- Docker: Used for containerizing Rails applications, ensuring consistency across environments and simplifying deployment and scalability issues.
- Git: Version control is essential for Rails development, with Git being the most popular system for tracking changes and managing code across collaborative projects.
Key Expertise Areas in Ruby on Rails Development
Ruby on Rails development encompasses various expertise areas that developers are typically expected to be proficient in to build and maintain robust web applications. Here are some of the essential skills and knowledge areas:
- MVC Architecture: Understanding the Model-View-Controller (MVC) pattern is crucial for Rails developers to organize code properly and enhance application maintainability.
- Ruby Programming: Proficiency in Ruby, the underlying language of Rails, is essential. This includes familiarity with Ruby syntax, constructs, and the Ruby standard library.
- Database Management: Expertise in relational databases like PostgreSQL or MySQL, including designing schemas, writing queries, and optimizing performance.
- Front-End Technologies: Knowledge of HTML, CSS, and JavaScript, along with frameworks like Bootstrap or Foundation and JavaScript libraries such as React or Vue.js.
- Testing and TDD: Experience with test-driven development (TDD) and tools like RSpec, MiniTest, and Capybara to write and maintain a suite of tests that ensure application reliability and bug-free code.
- RESTful API Design: Ability to design and implement APIs using Rails, understanding the principles of RESTful architecture to facilitate communication between applications.
- Version Control Systems: Proficiency with version control systems, primarily Git, for source code management, collaboration, and maintaining the development history.
- Deployment and Scaling: Skills in deploying Rails applications using services like Heroku, AWS, or Digital Ocean and knowledge of scaling applications to handle increased traffic and data.
- Security Practices: Understanding Rails security mechanisms to protect applications from common vulnerabilities such as SQL injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF).
- Rails Conventions and Best Practices: Familiarity with Rails conventions, the ability to use generators, and adherence to Rails best practices to enhance code quality and speed up development.
Need to Hire Ruby on Rails Developers?
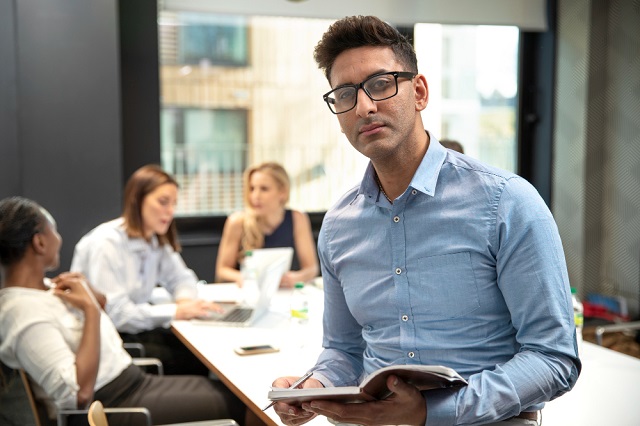
If you’re looking to expand your team with expert Ruby on Rails developers, Tier2Tek Staffing is ready to connect you with top-tier talent. Our specialized recruitment services ensure that you meet the perfect candidates for your development needs.