Full Stack Developer Interview Questions with Answers
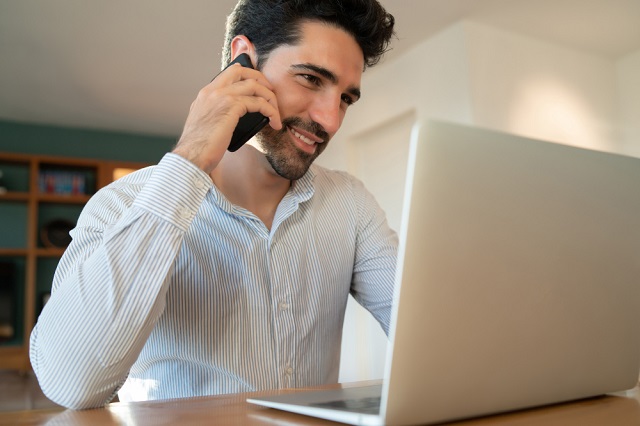
As a staffing company, we understand the importance of identifying highly skilled full stack developers who can efficiently handle both front-end and back-end development tasks. Full stack developers are integral to modern web development environments, bridging the gap between server-side and client-side programming.
To assist both hiring managers and job seekers in navigating the technical interview process, we’ve compiled a set of essential interview questions and their concise answers. These questions cover a range of topics from programming languages to database management, ensuring a thorough evaluation of candidates’ skills and knowledge.
What is a full stack developer?
A full stack developer is someone who can handle both the front-end and back-end portions of web applications. They work on the frontend (client side), backend (server side), database, and debugging of web applications or websites.
Explain the MVC architecture.
The Model-View-Controller (MVC) architecture divides an application into three interconnected parts. This is done to separate internal representations of information from the ways information is presented to and accepted from the user.
What is a RESTful API?
A RESTful API is an application program interface that uses HTTP requests to GET, PUT, POST and DELETE data. It is based on representational state transfer technology, commonly used in web services development.
Describe how you would create a simple webpage using HTML and CSS.
To create a simple webpage, start by writing HTML to structure the content with elements like <div>
, <p>
, and <h1>
. Use CSS to style these elements, setting properties like color
, font-size
, and margin
.
What are some security best practices for full stack development?
Some security best practices include using HTTPS, implementing proper authentication and authorization, securing data storage, and regularly updating and patching systems.
Can you explain the difference between SQL and NoSQL databases?
SQL databases are relational, table-based databases, while NoSQL databases can be document-oriented, key-value pairs, graph databases, or wide-column stores. The choice between them depends on the project requirements.
How do you ensure your website’s front-end is responsive?
To ensure a website’s front-end is responsive, use responsive design principles like flexible grids, flexible images, and CSS media queries to adapt the layout to different screen sizes and orientations.
What is version control and why is it important?
Version control is a system that records changes to a file or set of files over time so that specific versions can be recalled later. It is crucial for managing changes and collaboration in software development projects.
What is the purpose of a service worker in web development?
A service worker is a script that your browser runs in the background, separate from a web page, opening the door to features that don’t need a web page or user interaction. It’s primarily used to handle background synchronization and push notifications.
How do you handle CORS issues in web development?
CORS (Cross-Origin Resource Sharing) issues can be handled by configuring the server to include the appropriate CORS headers in its responses, specifically Access-Control-Allow-Origin
, to permit resources to be requested from another domain.
What frameworks have you used in your projects and why?
The choice of frameworks depends on project requirements. For example, React or Angular might be used for dynamic client-side applications, while Node.js is suitable for non-blocking, event-driven servers.
What are websockets and how do they work?
Websockets provide a way to open a two-way interactive communication session between the user’s browser and a server. This allows for real-time data transfer to and from the server without refreshing the page.
Describe how you would optimize a website’s performance.
Optimizing a website’s performance can involve minimizing file sizes, reducing server response times, using content delivery networks, and optimizing database queries.
Explain how you would handle multiple stylesheets for different webpages in a project.
Handling multiple stylesheets can be managed by using a CSS preprocessor like SASS or LESS, which allows for better organization with features like variables and mixins, or by linking specific stylesheets in the HTML depending on the page.
What is dependency injection and how is it used?
Dependency injection is a design pattern used in software development where an object receives other objects it depends on. This pattern allows for better modularity and makes the system easier to manage and test.
How do you ensure data integrity when sending data from the client to the server?
To ensure data integrity, use validation and sanitization processes on the client side before sending data, and verify or re-validate this data on the server side.
Describe the process of deploying a web application.
Deploying a web application involves several steps: testing the application, configuring the server, setting up databases, and potentially using automated deployment tools to manage the deployment process across different environments.
What is containerization in software development?
Containerization involves encapsulating software in a container with its own operating environment. It provides consistent, efficient, and secure deployments across different systems.
How do you manage state in a single-page application (SPA)?
State management in SPAs can be handled using libraries or frameworks like Redux or Context API in React, which provide structures that help manage state across components in a consistent manner.
Explain the role of HTTPS in web security.
HTTPS encrypts the data exchanged between a browser and a server, enhancing security by preventing eavesdropping, tampering, and forgery.
What is progressive enhancement in web development?
Progressive enhancement is a strategy for web design that emphasizes accessibility, semantic HTML markup, and external stylesheet and scripting technologies.
How do you handle database migrations in a project?
Database migrations are handled by writing scripts that alter the database structure, often using tools that track and apply migrations consistently across environments.
Describe your approach to testing and debugging a full stack application.
Testing involves several layers, including unit tests for individual components, integration tests for interactions between components, and end-to-end tests for the entire application. Debugging might involve using developer tools, debuggers, and logs.
What is API rate limiting and why is it used?
API rate limiting restricts the number of API requests a user can make in a certain period to prevent abuse and ensure service availability for all users.
Explain the concept of “single-page application” and its advantages.
A single-page application (SPA) is a web application that loads a single HTML page and dynamically updates that page as the user interacts with the app. Advantages include smoother user experience and reduced server load.
How do you ensure accessibility in web applications?
Ensuring accessibility involves following web accessibility guidelines, using semantic HTML, ensuring keyboard navigability, and providing alternative text for media.
What is the difference between client-side and server-side rendering?
Client-side rendering renders web pages directly in the browser, using JavaScript. Server-side rendering generates the full HTML for a page on the server in response to a user request. Each has different advantages for performance and SEO.
Describe how you handle updates to third-party libraries in your projects.
Handling updates to third-party libraries involves checking for updates regularly, testing the new versions in a development environment, and understanding the changes and their impact on the current project.
What tools do you use for monitoring and improving the performance of a web application?
Tools like Google Lighthouse, WebPageTest, and Chrome DevTools are used to monitor and improve the performance of web applications, providing insights into resource loading and execution times.
Common Technologies Used by Full Stack Developers
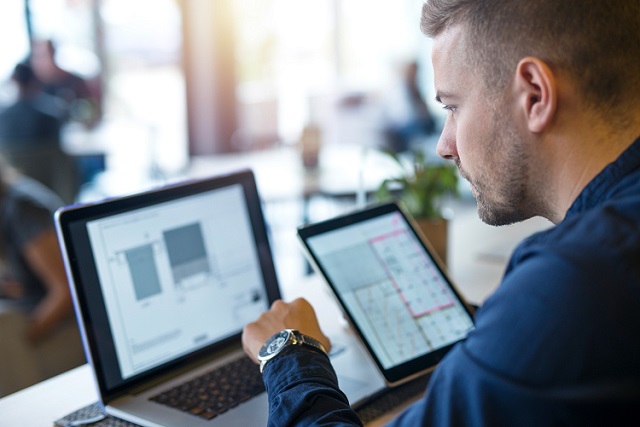
Full stack developers are proficient in a wide array of technologies to handle both the front-end and back-end aspects of web development. Here are some of the most commonly used technologies:
- Front-end Technologies:
- HTML/CSS: Building blocks of web design used to create pages and style them.
- JavaScript: Essential for dynamic interactions on web pages, and is supported by libraries and frameworks such as React, Angular, and Vue.js.
- Back-end Technologies:
- Node.js: Popular for full stack JavaScript development.
- Python: Widely used with frameworks like Django and Flask.
- Ruby: Known for its Rails framework, which is praised for its ease of use in building complex websites.
- Java: Utilized with Spring and Hibernate frameworks for robust back-end solutions.
- PHP: Commonly used with Laravel and CodeIgniter frameworks.
- Databases:
- SQL databases: Such as MySQL, PostgreSQL, and Microsoft SQL Server.
- NoSQL databases: Including MongoDB, Cassandra, and Redis.
- Version Control Systems:
- Git: Essential for tracking changes to code and collaboration.
- Development Tools and Environments:
- Docker: For containerization that simplifies deployment.
- Webpack and Babel: For bundling JavaScript files and transpiling ES6+ JavaScript into browser-compatible JavaScript.
- NPM/Yarn: Package managers for managing libraries and dependencies.
- Testing Frameworks:
- Jest: Popular for JavaScript testing.
- Mocha: Flexible testing framework for Node.js.
- Selenium: For automated browser testing.
- APIs:
- REST and GraphQL: For designing and consuming web services.
Key Expertise for Full Stack Developers
Full stack developers possess a diverse set of skills that span multiple layers of software development. Here’s a rundown of the common expertise that full stack developers typically require:
- Front-end Development:
- Proficiency in HTML, CSS, and JavaScript to build interactive and user-friendly interfaces.
- Familiarity with front-end frameworks and libraries like React, Angular, or Vue.js.
- Back-end Development:
- Ability to write server-side code using languages like Python, Ruby, Java, or Node.js.
- Experience with back-end frameworks such as Django, Rails, Spring, or Express.
- Database Management:
- Knowledge of both relational (SQL) and non-relational (NoSQL) databases.
- Skills in database design, management, and optimization techniques.
- API Design and Development:
- Competence in creating and integrating APIs using REST or GraphQL.
- Understanding of API authentication and authorization mechanisms like OAuth and JWT.
- Version Control and Code Collaboration:
- Proficiency with version control tools like Git.
- Experience in collaborating on large codebases within a team environment.
- Testing and Debugging:
- Skills in writing unit, integration, and end-to-end tests.
- Ability to debug and resolve issues across the full stack, from front-end UI to back-end and database interactions.
- DevOps and Deployment:
- Understanding of continuous integration and continuous deployment (CI/CD) pipelines.
- Familiarity with containerization tools like Docker and orchestration platforms like Kubernetes.
- Responsive and Adaptive Design:
- Experience with responsive web design techniques to ensure that applications work well on a variety of devices and screen sizes.
- Security Practices:
- Knowledge of web security practices to protect applications from common vulnerabilities like SQL injection, XSS, and CSRF attacks.
- Performance Optimization:
- Skills in optimizing application performance to enhance speed and efficiency, including techniques for front-end and back-end optimization.
Need to Hire Full Stack Developers?
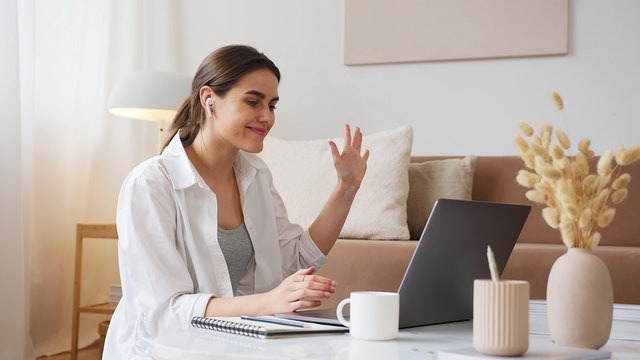
At Tier2Tek Staffing, we specialize in quickly connecting you with top-tier full stack developers, ensuring that you have the expertise needed to drive your projects forward.
Why Choose Us for Full Stack Developer Recruitment?
- Sourcing Speed: We pride ourselves on our rapid response times, quickly sourcing full stack developers to meet your project deadlines.
- Communication with Clients: Our commitment to open and ongoing communication ensures you’re always in the loop and satisfied with our service.
- Quality Candidates: We provide access to a pool of thoroughly vetted full stack developers, ensuring you receive the best match for your needs.
- Innovative Sourcing Strategies: We employ cutting-edge sourcing strategies to find and secure the most skilled and suitable full stack developers for your projects.