C# Interview Questions with Answers
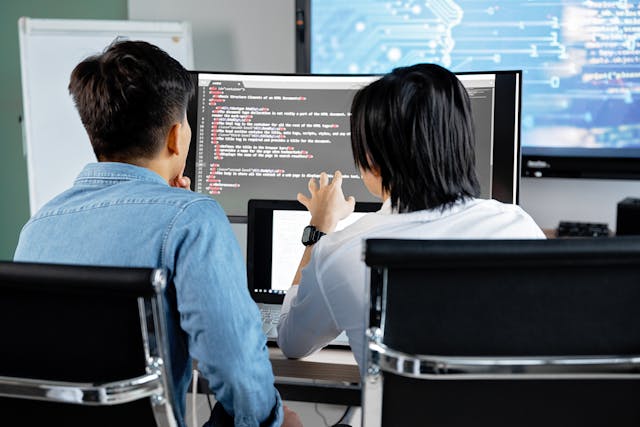
As a staffing company, it’s essential for both hiring managers and job seekers to be well-prepared for technical interviews, especially in the field of C#. C# is a versatile and widely used programming language that forms the backbone of many software applications, particularly those in the .NET ecosystem. To aid in the preparation process, we’ve compiled a list of top C# technical interview questions along with concise answers. These questions cover a range of topics from basic to advanced, ensuring a comprehensive understanding of the language and its capabilities.
What is C#?
C# is a modern, object-oriented programming language developed by Microsoft, which is used to build a variety of applications including web, mobile, and desktop applications.
Explain the basic structure of a C# program.
A typical C# program consists of a namespace declaration, a class, methods, attributes, and a Main method that serves as the entry point of the program.
What are namespaces in C#?
Namespaces in C# are used to organize large code projects by grouping related classes, interfaces, and other types into one scope, helping to control the scope of class and method names in larger programming projects.
What is an object in C#?
An object is an instance of a class that encapsulates data and functionality related to that data. Objects interact with one another through methods and properties.
What are the different types of constructors in C#?
C# supports several types of constructors including default constructors, parameterized constructors, copy constructors, and static constructors.
Explain inheritance in C#.
Inheritance is a fundamental concept of object-oriented programming that allows a class to inherit properties and methods from another class. In C#, classes can inherit from one base class and multiple interfaces.
What are interfaces in C#?
Interfaces are contracts that define the actions that an object can perform. An interface in C# only contains declarations of methods, properties, and events, without any implementation.
How does method overloading work in C#?
Method overloading in C# allows a class to have multiple methods with the same name but different parameters. This enables different implementations based on the parameter list.
What is encapsulation?
Encapsulation is an object-oriented programming concept that binds together the data and functions that manipulate the data and keeps both safe from outside interference and misuse.
What are properties in C#?
Properties in C# are special methods called accessors. They are used to read, write, or compute the values of private fields.
Explain the difference between managed and unmanaged code.
Managed code is executed by the CLR (Common Language Runtime) which provides services such as garbage collection and memory management. Unmanaged code is executed directly by the operating system outside the CLR environment.
What is an exception? How is it handled in C#?
An exception is a problem that arises during the execution of a program. In C#, exceptions are handled using try, catch, and finally blocks.
What are delegates in C#?
Delegates are type-safe pointers to methods. They are used to pass methods as arguments to other methods and can be used to define callback methods.
What are events in C#?
Events in C# are a way for a class to notify other classes or objects when something of interest occurs. The class that sends (or raises) the event is called the publisher and the classes that receive (or handle) the event are subscribers.
Explain polymorphism in C#.
Polymorphism allows methods to have the same name but behave differently based on the objects that call them. In C#, it can be achieved through overriding and overloading.
What is an abstract class?
An abstract class cannot be instantiated and is intended only to be a base class of other classes. It can contain both abstract methods (without body) and concrete methods (with implementation).
What is an assembly?
An assembly is a compiled code library used for deployment, versioning, and security in C#. There are two types: executable (.exe) and dynamic link library (.dll).
How does garbage collection work in C#?
Garbage collection in C# automatically releases memory occupied by objects that are no longer in use. It does this by periodically running and checking for objects that are no longer being referenced.
What is boxing and unboxing in C#?
Boxing is the process of converting a value type to an object type, thus storing it on the heap. Unboxing extracts the value type from the object.
What is LINQ and why is it used?
LINQ, or Language Integrated Query, is a set of technologies based on the integration of query capabilities directly into the C# language. LINQ is used to query various data sources such as databases, XML documents, and in-memory collections like lists or arrays.
Describe the role of the using
statement in C#.
The using
statement in C# is used to ensure the correct use of IDisposable objects. It automatically disposes of the object when the code block within the using
statement is left, helping manage resources like file streams or database connections efficiently.
What is the difference between const
and readonly
in C#?
const
is used for declaring constants that must be initialized at compile-time, whereas readonly
variables can be initialized at runtime inside the constructor. Once initialized, readonly
variables cannot be changed.
How do you manage memory in C#?
Memory in C# is managed automatically by the CLR via the garbage collector, which handles the allocation and release of memory. Developers can also use Dispose
method explicitly to release resources manually when they are no longer needed.
What is the partial
keyword used for?
The partial
keyword indicates that the definition of a class, struct, or interface can be split into multiple files. This is useful in large projects to allow multiple developers to work on the same class without file conflicts.
What is the difference between a class and a struct in C#?
In C#, classes are reference types and structs are value types. Structs are suitable for small data structures that contain primarily data that is not intended to be modified after the struct is created. Unlike classes, structs do not support inheritance.
Explain extension methods.
Extension methods allow developers to add new methods to existing types without modifying those types. These methods are static methods inside static classes, but they are called as if they were instance methods on the extended type.
What is the dynamic
keyword used for?
The dynamic
keyword in C# is used to bypass static type checking. Variables declared with dynamic
can store any type of value and the method and property invocations on these variables are resolved at runtime.
Describe the async
and await
keywords.
async
and await
are used for asynchronous programming in C#. async
is used to define a method as asynchronous, and await
is used within an async method to pause the execution of the method until a particular task is completed, without blocking the thread.
What are attributes in C#?
Attributes are metadata added to code elements such as classes, methods, and properties. They do not affect the execution of the code directly but can be used by the compiler and runtime to handle elements differently.
Explain the significance of the volatile
keyword.
The volatile
keyword is used to indicate that a field might be modified by multiple threads that are executing at the same time. The compiler and runtime are instructed not to cache the value of the field and always read it from its main location.
How can you handle multiple exceptions in C#?
In C#, multiple exceptions can be caught by specifying multiple catch blocks. Each catch block can handle a different type of exception, allowing for more precise control over exception handling.
What is the purpose of the nameof
expression?
The nameof
expression is used to obtain the simple (unqualified) string name of a variable, type, or member. It is useful for error-handling and logging purposes without hardcoding strings, reducing the potential for typos.
Common Technologies Used with C#
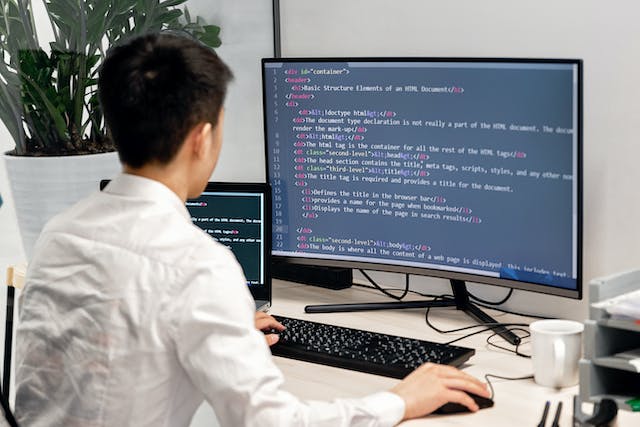
C# is a versatile programming language that integrates with various technologies to build robust applications. Some of the common technologies used with C# include:
- .NET Framework & .NET Core: These are platforms for building many different types of applications in C#, from web to desktop to mobile applications.
- ASP.NET: Used for building dynamic web sites, web applications, and web services. ASP.NET supports MVC (Model-View-Controller) architecture for web applications.
- Entity Framework: An ORM (Object-Relational Mapping) framework that lets developers work with databases by manipulating data as objects.
- Windows Presentation Foundation (WPF): A framework for developing graphical user interfaces in Windows-based applications.
- Xamarin: Used for developing mobile applications that can run on Android, iOS, and Windows Phone with a single code base.
- Microsoft SQL Server: A popular database often used with C# applications for data storage and management.
- LINQ (Language Integrated Query): Allows for querying various data sources such as databases, XML documents, and in-memory collections directly in C#.
- Blazor: A framework for building interactive client-side web UIs with C# instead of JavaScript.
- SignalR: A library for adding real-time web functionalities to applications, enabling server-side code to push content to clients instantly.
Key Expertise Areas in C# Development
C# developers typically possess expertise in several critical areas that enable them to build and maintain robust applications. Some of the key expertise areas include:
- Object-Oriented Programming (OOP): Profound understanding of concepts like inheritance, encapsulation, polymorphism, and abstraction.
- Understanding of .NET and .NET Core Frameworks: Deep knowledge of the frameworks that provide a comprehensive and consistent programming model for building applications.
- Database Management: Skills in handling databases, particularly with Entity Framework and SQL, to perform CRUD operations (Create, Read, Update, Delete) and manage data effectively.
- Web Development: Expertise in ASP.NET for creating dynamic web pages and web applications. This includes both ASP.NET MVC and newer technologies like Blazor.
- Desktop Application Development: Experience with Windows Forms (WinForms) and Windows Presentation Foundation (WPF) for creating user-friendly desktop applications.
- Mobile Application Development: Knowledge of Xamarin for creating mobile applications that can operate on multiple platforms using a single codebase.
- API Development: Ability to create and consume APIs efficiently, which is crucial for modern application architecture involving web services.
- Unit Testing and Test-Driven Development (TDD): Skills in testing frameworks like NUnit and xUnit to ensure code reliability and functionality through rigorous testing practices.
- Asynchronous Programming and Multithreading: Competence in handling asynchronous programming patterns and multithreading to enhance application performance and responsiveness.
- Security Practices: Understanding of common security concerns and best practices in C# programming to protect data and ensure application integrity.
Need to Hire C# Developers?
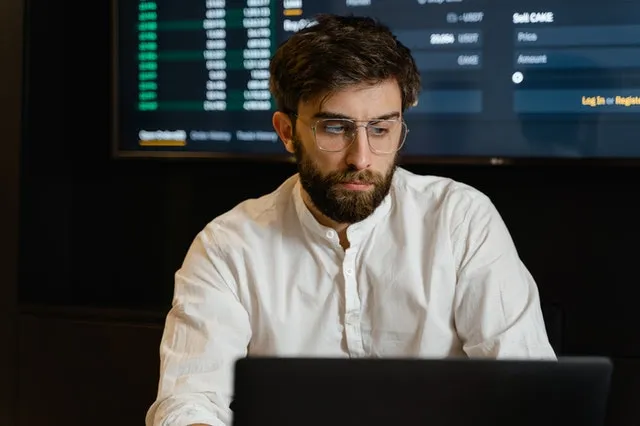
At Tier2Tek Staffing, we specialize in quickly connecting you with top-tier C# developers. Whether you’re scaling up a project or need specialized skills, we have the expertise to fulfill your hiring needs efficiently.