PHP Developer Interview Questions with Answers
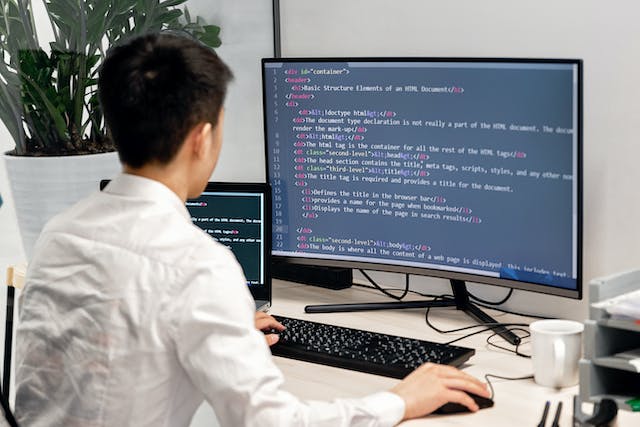
For hiring managers and job seekers alike, the interview process for a PHP Developer position is crucial for finding the right candidate who not only understands the technical aspects of PHP but also possesses the ability to apply these skills effectively. We’ve compiled targeted interview questions along with concise and straightforward answers to help assess the capabilities of potential PHP developers. These questions cover a range of topics from basic syntax and functions, to more complex problem-solving scenarios.
1. What are the main error types in PHP, and how do they differ?
Answer: The main error types in PHP are notices, warnings, and fatal errors. Notices are minor issues that do not stop the script from running. Warnings are more serious but the script will still run. Fatal errors cause the script to stop execution.
2. Explain the use of sessions in PHP.
Answer: Sessions in PHP are used to store information about a user across multiple pages. It helps maintain data across subsequent accesses, useful for building features like user login systems.
3. What is the difference between GET
and POST
methods in form submission?
Answer: GET
appends data to the URL and is visible, making it less secure and limited in data length. POST
sends data as part of the request body, offering more security and capacity for larger data volumes.
4. How can you prevent SQL injection in PHP?
Answer: SQL injection can be prevented by using prepared statements with PDO (PHP Data Objects) or MySQLi, which automatically handle escaping special characters thus securing database queries.
5. Describe what a PDO is in PHP.
Answer: PDO stands for PHP Data Objects, a database access layer providing a uniform method of access to multiple databases.
6. What does the include
statement do in PHP?
Answer: The include
statement takes all the text in a specified file and copies it into the file that uses the include
statement. It’s used to insert the contents of one PHP file into another PHP file before the server executes it.
7. What are PHP Magic Methods?
Answer: PHP magic methods are special functions that have specific names starting with double underscores (__
) like __construct()
, __destruct()
, __call()
, etc. They are triggered in response to particular PHP events.
8. What is the purpose of the __construct()
method?
Answer: The __construct()
method is a magic method that automatically initializes an object when it is created, often used to set up initial object properties.
9. How do you declare a constant in PHP?
Answer: Constants in PHP are defined using the define()
function or the const
keyword. Once set, their values cannot be changed during script execution.
10. What are traits in PHP?
Answer: Traits are a mechanism for code reuse in PHP. Traits can have methods that can be inserted into a class in a similar way to classes.
11. Explain namespacing in PHP and its benefits.
Answer: Namespaces in PHP are used to encapsulate items like classes, interfaces, functions, and constants. This helps prevent name collisions between code pieces from different libraries or applications.
12. What is the purpose of the final
keyword in PHP?
Answer: The final
keyword prevents child classes from overriding a method by prefixing the definition with final
. If the class itself is being defined final, then it cannot be extended.
13. What does ===
operator do in PHP?
Answer: The ===
operator, also known as the identity operator, checks if the value and the type of two variables are identical.
14. How can you increase the execution time of a PHP script?
Answer: The execution time can be increased by changing the max_execution_time
directive in the php.ini file, or by calling set_time_limit()
function in the script.
15. What is the difference between echo
and print
in PHP?
Answer: Both echo
and print
are used to output data to the screen. echo
has no return value, is slightly faster and can take multiple parameters, while print
has a return value of 1 and can only take one argument.
16. How do you check if a variable is an array in PHP?
Answer: Use the is_array()
function to check whether a variable is an array.
17. What is the difference between unset()
and unlink()
?
Answer: unset()
destroys a given variable, while unlink()
deletes a file we are referring to from the file system.
18. How do you start and destroy a session in PHP?
Answer: A session is started with the session_start()
function and destroyed with session_destroy()
function.
19. What are interfaces in PHP and how are they used?
Answer: Interfaces in PHP define public methods that a class must implement without implementing the methods. They ensure a class adheres to a particular contract.
20. Explain inheritance in PHP.
Answer: Inheritance is a principle where a new class (child class) is based on an existing class (parent class), inheriting all public and protected properties and methods.
21. How can you prevent Cross-site Scripting (XSS) in PHP?
Answer: XSS can be prevented by sanitizing user input, especially data that is output to the browser. This can involve stripping tags, encoding or validating inputs.
22. What is the implode()
function in PHP?
Answer: The implode()
function joins elements of an array into a single string.
23. Describe the use of header() function in PHP.
Answer: The header()
function sends a raw HTTP header to a client. It’s often used to redirect users to another page.
24. What are constructors and destructors in PHP?
Answer: Constructors are methods that are automatically called upon the creation of an object. Destructors are called when an object is destructed or the script ends.
25. Explain the difference between array_merge()
and array_combine()
.
Answer: array_merge()
merges one or more arrays into one array, while array_combine()
creates a new array, using one array for keys and another for its values.
26. How does foreach
loop work in PHP?
Answer: The foreach
loop iterates over elements of an array or properties of an object.
27. What are anonymous functions in PHP?
Answer: Anonymous functions, also known as closures, allow the creation of functions which have no specified name. They are most useful as the value of callback parameters.
28. What is strtotime()
used for in PHP?
Answer: strtotime()
is used to convert an English textual datetime description into a Unix timestamp.
29. How can you handle exceptions in PHP?
Answer: Exceptions in PHP can be handled using the try
, catch
, and finally
statements. Code that may throw an exception is put inside the try
block and the exception is caught in the catch
block.
30. Explain the difference between include
, include_once
, require
and require_once
.
Answer: include
and require
are used to insert the contents of one PHP file into another PHP file; include
does not stop the script execution on error, while require
does. Adding _once
prevents files from being included or required more than once.
Common Technologies Used by PHP Developers
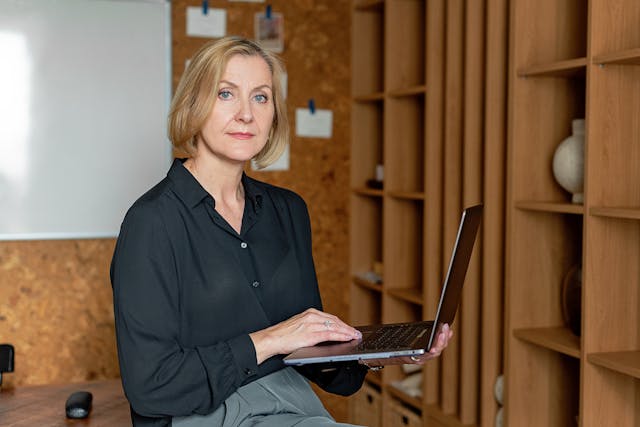
PHP developers typically work with a variety of technologies to build and maintain dynamic and interactive web applications. Here are some of the most common technologies associated with PHP development:
- MySQL: A popular relational database management system often used with PHP for database functionality.
- Apache: A widely used web server software that PHP developers commonly deploy alongside PHP to serve web pages.
- Laravel: A modern PHP framework for web applications that provides a structured and standardized way to build robust web applications.
- Symfony: Another PHP framework that provides components and tools to developers for building complex web applications more efficiently.
- Composer: A dependency management tool for PHP, allowing developers to manage libraries that their projects depend on.
- JavaScript: Often used alongside PHP to enhance the interactivity of web pages. Libraries like jQuery are commonly used.
- HTML/CSS: Essential for web development, used to structure content and style it on the web pages that PHP serves.
- Git: Version control system commonly used for managing PHP project code, especially in team environments.
- Docker: Used to containerize PHP applications, ensuring they run consistently across different computing environments.
- RESTful APIs: PHP is often used to develop RESTful services because of its simplicity and built-in web capabilities.
Key Expertise for PHP Developers
PHP developers are expected to have a range of skills and expertise that enable them to handle various aspects of web development efficiently. Here are some key areas of expertise commonly required for PHP developers:
- Core PHP Programming: Proficiency in the syntax, semantics, and runtime behavior of PHP.
- Object-Oriented Programming (OOP): Understanding and applying OOP principles in PHP to create modular, reusable, and maintainable code.
- Database Management: Skills in SQL and experience with database systems like MySQL, PostgreSQL, or MariaDB, including designing, querying, and optimizing databases.
- Framework Proficiency: Knowledge of PHP frameworks such as Laravel, Symfony, or CodeIgniter, which help in rapid development and maintaining standard coding practices.
- Front-end Technologies: Familiarity with HTML, CSS, JavaScript, and front-end frameworks like Angular, React, or Vue.js to manage the application’s user interface.
- Version Control Systems: Experience with tools like Git to manage code versions and collaborate with other developers effectively.
- Security Practices: Understanding of web security practices to prevent common vulnerabilities such as SQL injections, XSS attacks, and CSRF attacks.
- API Integration and Development: Ability to integrate third-party services through APIs and develop RESTful APIs to allow others to interface with the application.
- Testing and Debugging: Skills in using testing frameworks like PHPUnit and debugging tools to ensure the application runs smoothly and efficiently.
- Performance Optimization: Knowledge of techniques to enhance the performance of PHP applications, such as caching mechanisms, code profiling, and query optimization.
Need to Hire PHP Developers?
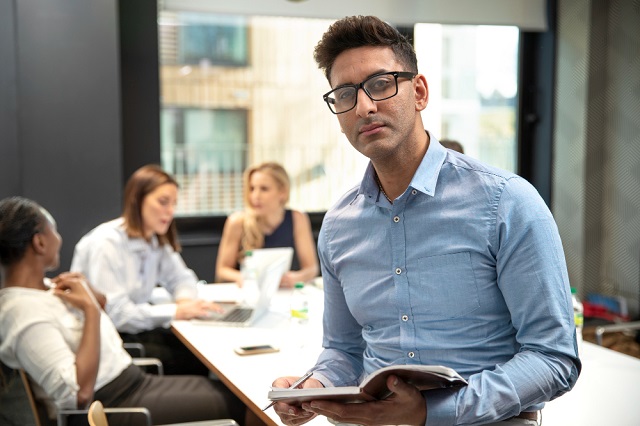
If you’re looking to expand your team with skilled PHP developers, Tier2Tek Staffing can connect you with top talent efficiently and effectively. Our expertise ensures that we understand your specific requirements and help you find the perfect match.
- Sourcing Speed: We quickly deliver a list of qualified PHP developers, minimizing your hiring downtime.
- Communication with Clients: You’ll experience seamless communication, ensuring all your staffing needs are met promptly and accurately.
- Quality Candidates: We provide access to a vetted pool of PHP developers, ensuring each candidate meets your high standards.
- Innovative Sourcing Strategies: Our team employs cutting-edge strategies to source the best talent, tailored to the dynamic needs of PHP development.