JavaScript Developer Interview Questions with Answers
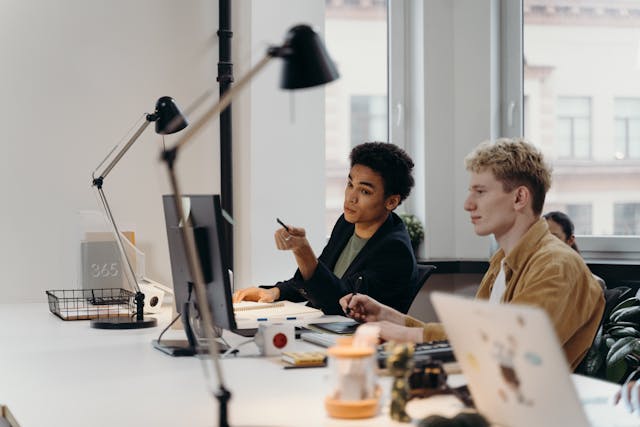
When hiring JavaScript developers, it’s crucial to assess their technical skills and problem-solving abilities effectively. The following set of questions is designed to help hiring managers and job seekers alike understand the depth of knowledge required in JavaScript development. These questions cover a range of topics from basic syntax and functionalities to advanced programming concepts and patterns.
What is JavaScript and why is it important in web development?
JavaScript is a programming language that allows you to implement complex features on web pages. It is essential for adding interactive elements such as games, animations, and form validations, making websites more dynamic and user-friendly.
How do you declare a variable in JavaScript?
Variables in JavaScript can be declared using var
, let
, or const
. let
is block-scoped, var
is function-scoped, and const
is used for variables whose values are not intended to change.
What are JavaScript Data Types?
JavaScript includes data types such as Number, String, Boolean, Null, Undefined, Object, and Symbol. Each type serves a different purpose, like storing text, numeric values, or complex objects.
Explain the difference between ==
and ===
in JavaScript.
==
is the equality operator that performs type coercion if the variables on either side are different types. ===
is the strict equality operator that does not perform type coercion and only returns true if both the value and type are identical.
What are template literals in JavaScript?
Template literals are string literals allowing embedded expressions, denoted by backticks (
). They can contain placeholders indicated by
${expression}`, which makes it easier to build dynamic strings.
Describe let
, var
, and const
.
var
declarations are globally scoped or function-scoped and can be redeclared and updated. let
is block-scoped and cannot be redeclared within its scope but can be updated. const
is block-scoped, cannot be updated or redeclared, and must be initialized at the time of declaration.
What are arrow functions?
Arrow functions provide a concise syntax for writing function expressions. They do not have their own this
, do not have arguments
, cannot be used as constructors, and are best suited for non-method functions.
Can you explain how this
keyword works in JavaScript?
The this
keyword refers to the object it belongs to. In a method, this
refers to the owner object. Alone, this
refers to the global object. In a function, this
refers to the global object or undefined in strict mode. In an event, this
refers to the element that received the event.
What is a closure in JavaScript?
A closure is a feature where an inner function has access to the outer (enclosing) function’s variables—a scope chain. The closure has three scope chains: it has access to its own scope, the outer function’s variables, and the global variables.
Explain the use of the new
keyword in JavaScript.
The new
keyword is used to create an instance of an object that has a constructor function. It creates a new empty object and binds this
to the new object, allowing you to add properties and methods to it.
What is event bubbling and capturing?
Event bubbling and capturing are two ways of event propagation in the HTML DOM API when an event occurs in an element inside another element. Bubbling means the event is first captured and handled by the innermost element and then propagated to outer elements. Capturing is the opposite.
How do you create a class in JavaScript?
Classes in JavaScript are primarily syntactic sugar over JavaScript’s existing prototype-based inheritance and are introduced in ES6. Here is how you define a class:
javascriptCopy codeclass Rectangle {
constructor(height, width) {
this.height = height;
this.width = width;
}
}
What is the purpose of JavaScript async/await keywords?
The async/await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains. async
makes a function return a Promise, and await
makes a function wait for a Promise.
How does JavaScript handle asynchronous programming?
JavaScript handles asynchronous programming through callbacks, promises, and async/await. These features allow JavaScript to perform non-blocking operations, such as loading data from a server.
What are Promises in JavaScript?
A Promise is an object representing the eventual completion or failure of an asynchronous operation. It allows you to associate handlers with an asynchronous action’s eventual success value or failure reason.
Explain the Module pattern in JavaScript.
The Module pattern is used to encapsulate a group of related functions or variables into a single object or module. This pattern promotes cleaner, more readable code and reduces namespace pollution by preventing
the exposure of private properties and methods to the global scope. Here’s a basic example:
javascriptCopy codevar Module = (function () {
var privateMethod = function () {
// Private
};
return {
publicMethod: function () {
// Public
}
};
})();
What is event delegation in JavaScript?
Event delegation is a technique where a single event listener is attached to a parent element instead of multiple listeners on individual child elements. It utilizes the event bubbling phase to handle events at a higher level in the DOM.
How do you handle exceptions in JavaScript?
Exceptions in JavaScript are handled using the try...catch
statement. Code that might throw an exception is placed inside the try
block, and code to execute in case of an exception is placed in the catch
block.
What are JavaScript Generators?
Generators are functions that can be exited and later re-entered, with their context (variable bindings) saved across re-entrances. Generators are defined using the function*
syntax and use the yield
keyword.
What is the difference between null
and undefined
in JavaScript?
null
is an assignment value representing no value, while undefined
signifies that a variable has been declared but has not yet been assigned a value. Both represent empty values in different ways.
What are the different ways to handle asynchronous operations in JavaScript?
JavaScript offers several methods for handling asynchronous operations, such as callbacks, promises, async/await, and observables (with libraries like RxJS). Each has different use cases and benefits.
Describe the Prototype Chain in JavaScript.
In JavaScript, each object has a private property which holds a link to another object called its prototype. This prototype has its own prototype, forming a chain ending at null
. Properties and methods are looked up along this chain.
What is the Event Loop in JavaScript?
The event loop is a mechanism that handles executing multiple chunks of code over time, by managing a queue of messages to be processed. It allows JavaScript to perform non-blocking operations despite being single-threaded.
Explain JavaScript’s single-threaded nature.
JavaScript is single-threaded, meaning it can only execute one command at a time. The call stack and event loop allow JavaScript to perform asynchronous tasks by handling function calls and event handling in an orderly manner.
How do you prevent code blocks from leaking scope in JavaScript?
To prevent scope leakage in JavaScript, use block-scoped variables (let
or const
) instead of var
. This restricts variable access to the block in which they are defined, preventing unintended interactions with other parts of the code.
What is JSON and how does JavaScript use it?
JSON (JavaScript Object Notation) is a lightweight data interchange format. JavaScript uses JSON to exchange data with a server or to store information. It is easy to read and write and can be parsed and generated by many programming languages.
Explain the reduce
method in JavaScript arrays.
The reduce
method processes each element of an array with a specified reducer function and returns a single output value. It is useful for aggregating or accumulating values based on elements of an array.
javascriptCopy codeconst numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
What are Service Workers and when might you use them?
Service Workers are scripts that run in the background, separate from the web page, and enable capabilities such as push notifications and background sync. They are primarily used to create effective offline experiences and intercept network requests to provide programmatic control over cache.
Common Technologies Used by JavaScript Developers
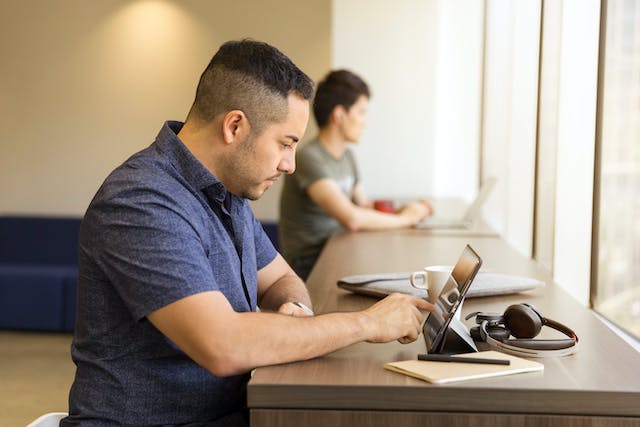
JavaScript developers commonly utilize a variety of technologies to build and enhance web applications. These include:
- Node.js: This JavaScript runtime allows developers to run JavaScript on the server side, enabling the development of scalable network applications.
- React.js: A popular JavaScript library for building user interfaces, particularly single-page applications where data responds to user actions without reloading the page.
- Angular: A platform and framework for building client-side applications, providing a comprehensive solution for developing efficient and sophisticated single-page apps.
- Vue.js: A progressive JavaScript framework used for building UIs and single-page applications. It is designed to be incrementally adoptable.
- TypeScript: A superset of JavaScript that adds static types to the language, helping to catch errors early and improve the readability and maintainability of the code.
- jQuery: Although its usage has declined with the rise of modern frameworks, jQuery is a fast and concise JavaScript library that simplifies HTML document traversing, event handling, and animation.
- Webpack: A module bundler for JavaScript applications that packages all the necessary assets, including JavaScript, images, and CSS, into one or more bundles.
- ESLint: A pluggable linting utility for JavaScript and JSX, helping developers follow consistent style guidelines and avoid errors in their JavaScript code.
- Babel: A JavaScript compiler that allows developers to use next generation JavaScript, today. It converts ECMAScript 2015+ code into a backwards compatible version for current and older browsers or environments.
Key Expertise Areas for JavaScript Developers
JavaScript developers typically excel in several core areas of expertise that enable them to create dynamic and responsive web applications. These areas include:
- Front-End Development: Proficiency in HTML, CSS, and front-end frameworks such as React.js, Angular, and Vue.js to build interactive user interfaces.
- Back-End Development: Knowledge of server-side programming, especially using Node.js, to handle database operations, user authentication, and server logic.
- Responsive Design: Skills in creating applications that work seamlessly on various devices and screen sizes using responsive design techniques.
- State Management: Expertise in managing state in complex applications using libraries and tools like Redux or MobX, especially important in React development.
- RESTful Services and APIs: Ability to create and integrate RESTful services and work with external APIs to fetch, display, and manipulate data.
- Version Control Systems: Proficiency in using version control systems like Git, which is crucial for collaboration and maintaining the codebase in development teams.
- Testing and Debugging: Experience in testing frameworks such as Jest or Mocha and debugging tools to ensure the reliability and performance of applications.
- Performance Optimization: Skills in optimizing application performance to improve loading times and responsiveness by using techniques like lazy loading, effective caching, and minimizing resource usage.
- Security Practices: Knowledge of security best practices, such as preventing XSS and CSRF attacks and handling data securely.
- Agile Methodologies: Familiarity with Agile development practices and tools to enhance collaboration and adaptability in fast-paced development environments.
Need to Hire JavaScript Developers?
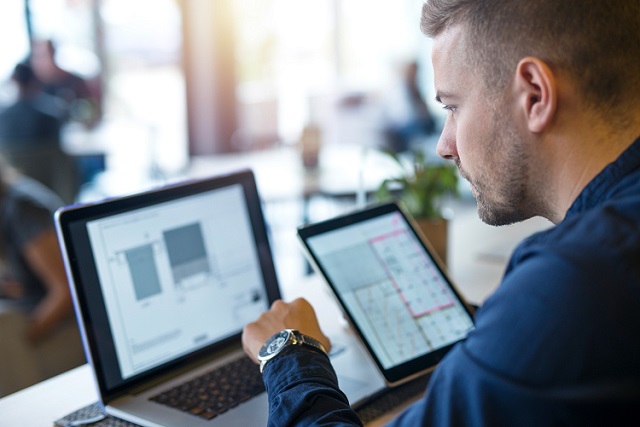
If you’re looking to expand your team with skilled JavaScript developers, Tier2Tek Staffing can swiftly connect you with top-tier talent suited to your project needs.
Why Choose Us?
- Sourcing Speed: We rapidly source JavaScript developers, ensuring your positions are filled without delay.
- Communication with Clients: Our team maintains open and continuous communication, keeping you informed throughout the hiring process.
- Quality Candidates: We provide only the most proficient and vetted JavaScript developers to meet your technical requirements.
- Innovative Sourcing Strategies: We employ cutting-edge sourcing techniques to find exceptional talent for JavaScript development roles.