Java Developer Interview Questions with Answers
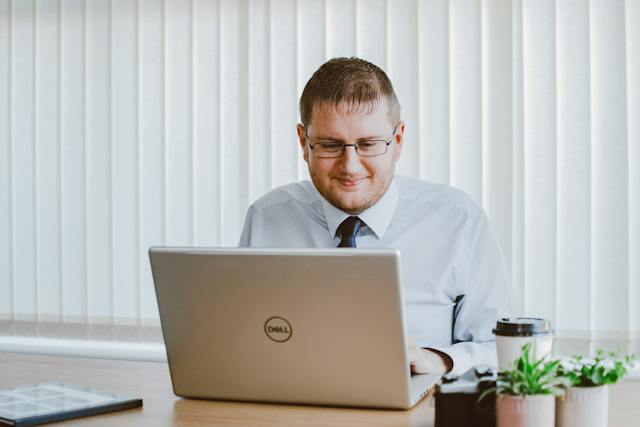
When hiring a Java Developer, it’s crucial to assess both their technical prowess and their ability to practically apply Java concepts in real-world scenarios. This guide is designed for hiring managers aiming to select the most competent candidates, as well as for job seekers preparing to showcase their Java expertise. Below are key technical interview questions with concise and simplified answers to help evaluate or demonstrate proficiency in Java development.
What is the Java Virtual Machine (JVM) and why is it important?
The JVM is a platform that allows Java programs to run on different hardware and operating systems without modification. It is important because it provides the environment for executing Java bytecode, making Java applications portable and secure.
What are the differences between JDK, JRE, and JVM?
- JVM (Java Virtual Machine): Executes Java bytecode and provides the runtime environment.
- JRE (Java Runtime Environment): Includes the JVM and libraries Java applications need to run.
- JDK (Java Development Kit): Contains the JRE and development tools like the compiler and debugger needed to develop Java applications.
Explain the concept of ‘inheritance’ in Java.
Inheritance is a mechanism where a new class derives attributes and methods from an existing class. In Java, this helps in code reusability and establishing a hierarchical classification of classes that share common traits.
What is exception handling in Java?
Exception handling is a mechanism to handle runtime errors, allowing the program to run without interruption. It’s done using try
, catch
, and finally
blocks to catch and handle exceptions effectively.
How does Java achieve platform independence?
Java achieves platform independence through its use of the JVM, which allows the same Java program bytecode to be executed on any system that has a compatible JVM, regardless of the underlying hardware and operating system.
What is the difference between ==
and .equals()
in Java?
==
: Checks if two references point to the exact same object in memory..equals()
: Method that checks if two objects are logically equal as defined by the class’sequals()
method.
Can you explain the concept of multithreading in Java?
Multithreading in Java is a feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU. Each part of such a program is called a thread, and threads share the same process stack and system resources but can run independently.
What is garbage collection in Java?
Garbage collection is the process by which Java programs perform automatic memory management. Java removes objects that are no longer being used by a program to free up memory resources.
What is the use of the static
keyword in Java?
The static
keyword in Java is used to indicate that a particular field or method belongs to the class, rather than instances of the class. This means static members can be accessed without creating an instance of the class.
What is a Java interface?
A Java interface is a reference type in Java, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types. Interfaces are abstract, so they cannot contain concrete methods (methods with bodies) until Java 8 introduced default and static methods.
Describe the final
keyword in Java and its impact.
The final
keyword in Java can be used in several contexts:
- final class: Prevents the class from being subclassed.
- final method: Prevents the method from being overridden by subclasses.
- final variable: Variables declared with
final
must be initialized only once and their value cannot be changed.
What is synchronization in Java?
Synchronization in Java is the tool that controls the access of multiple threads to any shared resource. It is used to prevent thread interference and consistency problems.
Explain how Java handles memory leaks.
Java manages memory through garbage collection, which automatically reclaims memory used by objects that are no longer reachable in the program. However, memory leaks can still occur if references to objects are held unnecessarily, preventing the garbage collector from reclaiming that memory.
How does the try-with-resources
statement work in Java?
The try-with-resources
statement simplifies the management of resources like files or sockets. Resources specified in the try clause are automatically closed after the statement execution, regardless of whether the execution completes normally or due to an exception.
What are Java annotations?
Java annotations are a form of metadata that provide data about a program but are not part of the program itself. Annotations have no direct effect on the operation of the code they annotate. They are used by the compiler and at runtime by the application.
Explain the difference between overloading and overriding in Java.
- Overloading: Occurs when two or more methods in one class have the same method name but different parameters. It is a way to create multiple methods with the same name, but different implementations.
- Overriding: Refers to a subclass providing a specific implementation of a method that is already provided by its superclass.
What is the difference between an abstract class and an interface in Java?
- Abstract class: Can have both abstract and concrete methods. It is used to share a common design without providing a full implementation of all its methods.
- Interface: Typically contains abstract methods that must be implemented by classes that implement the interface. Java 8 and later allow default and static methods in interfaces.
What is polymorphism in Java?
Polymorphism in Java is the ability of an object to take on many forms. It refers to the ability to perform a certain action in different ways. In Java, this is achieved by method overriding and method overloading.
Explain the Singleton design pattern in Java.
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to this instance. It is typically implemented by making the class constructor private and creating a static method that returns a reference to the instance.
What are generics in Java?
Generics enable types (classes and interfaces) to be parameters when defining classes, interfaces, and methods. This provides stronger type checks at compile time and can eliminate the need for casting, which can help in reducing runtime errors.
What is the volatile
keyword in Java?
The volatile
keyword in Java is used to indicate that a variable’s value will be modified by different threads. Declaring a Java variable as volatile
ensures that the value of the variable is always read from the main memory and not from the thread’s private cache.
Describe the process of object serialization in Java.
Object serialization in Java is the process of converting an object into a byte stream, thereby enabling the object to be transmitted over a network or stored in a file or database. It is typically used in networking, as data objects need to be transmitted between the client and the server.
Explain the concept of Java reflection.
Java reflection is a feature that allows examination or modification of the runtime behavior of applications. With reflection, you can inspect classes, interfaces, fields, and methods at runtime, without knowing the names of the classes, methods, etc., at compile time.
How does Java handle dependency injection?
Dependency injection in Java is a technique whereby one object supplies the dependencies of another object. It is a fundamental aspect of the Spring framework, which can manage components and their dependencies through either XML configuration or annotations.
What is the Java Memory Model?
The Java Memory Model (JMM) specifies how threads interact through memory and what behaviors are allowed in concurrent execution. It defines the interaction between Java threads and memory and ensures visibility of field changes to other threads, which is crucial for reliable and efficient multithreading.
How does the Java compiler handle type erasure in generics?
Type erasure in Java generics means that the generic type information is not retained at runtime. The Java compiler uses type erasure to enforce type constraints only at compile time and erases the element type information once compilation is completed. This means that the compiled code contains ordinary classes, interfaces, and methods without any type parameters.
What are lambda expressions in Java, and how are they used?
Lambda expressions are a feature introduced in Java 8 that enable you to treat functionality as a method argument or code as data. They provide a clear and concise way to implement functional interfaces using an expression. They are extensively used in functional programming, stream API, and to provide implementations of functional interfaces.
What is a deadlock in Java and how can you avoid it?
A deadlock in Java occurs when two or more threads are blocked forever, each waiting for the other to release a resource they need. Deadlocks can be avoided by ensuring that all locks are always acquired and released in the same order by any thread that needs them.
Explain the difference between Checked and Unchecked exceptions in Java.
- Checked exceptions: These are exceptions that are checked at compile-time. It means if a method is throwing a checked exception then it should either handle the exception using
try-catch
block or it must declare it using thethrows
keyword. Examples includeIOException
,SQLException
. - Unchecked exceptions: These are the exceptions that are not checked at compile-time. In other words, the compiler does not require methods to catch or to declare it. Unchecked exceptions are mostly caused by poor programming practices. Examples include
NullPointerException
,ArrayIndexOutOfBoundsException
.
What are the benefits of using Spring framework in Java?
Spring framework provides comprehensive infrastructure support for developing Java applications. Benefits include:
- Dependency Injection: Helps in gluing these classes together and at the same time keeping them independent.
- Aspect-Oriented Programming: Supports the separation of cross-cutting concerns, such as transaction management.
- MVC Framework: An HTTP and Servlet based framework providing hooks for customization and extension.
- Transaction Management: Unifies several transaction management APIs and coordinates transactions for Java objects.
How do you handle memory leaks in Java applications?
Handling memory leaks in Java involves:
- Identifying the root cause of the leak by profiling the application or analyzing heap dumps using tools like VisualVM, Eclipse Memory Analyzer, etc.
- Fixing improper use of static fields, misuse of Contexts in Android, or generally objects that live longer than necessary.
- Proper implementation of the
hashCode()
andequals()
methods in classes, especially when used in collections likeHashMap
andHashSet
.
What is the Stream API in Java? Provide a brief overview.
The Stream API, introduced in Java 8, is used to process collections of objects in a declarative way. It allows you to perform complex, aggregate operations on data sets efficiently with less code. The API supports sequential as well as parallel execution, making it possible to perform bulk operations on data asynchronously.
Explain the use of the transient
keyword in Java.
The transient
keyword in Java is used to indicate that a field should not be serialized — that is, its state should not be written to the byte stream during serialization. Fields declared as transient are not persisted when an object is saved to a persistent storage area.
Discuss the role of the implements
keyword in Java.
The implements
keyword in Java is used by a class to implement an interface. This means providing concrete implementations for all abstract methods declared in the interface. A class can implement multiple interfaces, distinguishing Java from languages with single inheritance but no multiple inheritance of classes.
What is method chaining in Java and how is it implemented?
Method chaining is a design pattern where several methods are called in a single line of code through a sequence of method calls returning the current object itself. This pattern is commonly used in the builder pattern, which simplifies object construction through fluent interfaces.
How can you ensure thread safety in Java?
Ensuring thread safety in Java can be achieved by:
- Using synchronized methods or blocks to ensure that only one thread can access a resource at a time.
- Employing thread-safe classes from the Java Collections framework like
Vector
,Hashtable
, orConcurrentHashMap
. - Utilizing atomic classes like
AtomicInteger
orAtomicReference
from thejava.util.concurrent.atomic
package for operations that involve compound actions.
What is a memory barrier in Java?
A memory barrier is a construct used by the JVM to prevent memory read/write reordering by the compiler and ensures visibility of changes to variables across threads. It is a crucial part of synchronization primitives like volatile
, which rely on memory barriers to ensure that changes to a variable are immediately visible to other threads.
Common Technologies Used by Java Developers
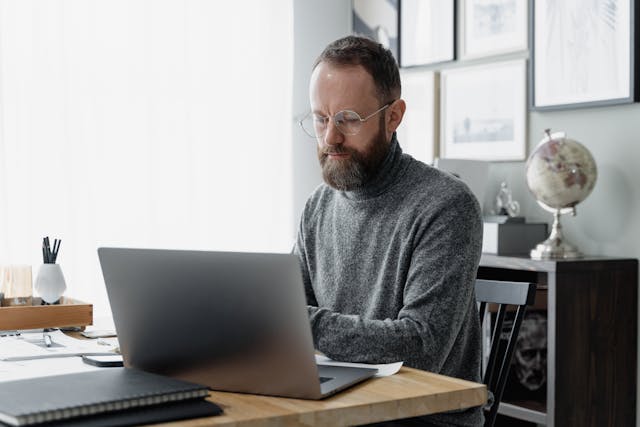
Java Developers typically utilize a range of technologies and tools to build efficient applications and services. Some of the most common technologies include:
- Java Development Kit (JDK): The essential toolkit for any Java developer which includes the Java Runtime Environment (JRE), an interpreter/loader (Java), a compiler (javac), an archiver (jar), a documentation generator (javadoc), and other tools necessary for Java development.
- Integrated Development Environments (IDEs): Tools like IntelliJ IDEA, Eclipse, and NetBeans provide powerful platforms for coding, debugging, and testing Java applications.
- Spring Framework: A popular framework for building enterprise applications, offering comprehensive infrastructure support for developing Java apps.
- Hibernate: An object-relational mapping (ORM) tool for database operations, widely used in conjunction with JPA (Java Persistence API).
- Apache Maven and Gradle: These are build automation tools used for managing project dependencies and automating builds.
- JUnit: A framework for performing unit testing to ensure that the code works as expected.
- Jenkins: An automation server used for continuous integration and continuous delivery, enhancing the development process.
- Git: Version control system used to manage source code history, collaborating on code changes, and maintaining a history of project changes.
Key Expertise Areas for Java Developers
Java Developers are expected to have a wide range of expertise to effectively design, develop, and manage software applications. Some of the most common areas of expertise include:
- Core Java Programming: Proficiency in Java fundamentals, such as object-oriented programming, interfaces, threads, and exception handling.
- Web Technologies: Knowledge of web protocols and web development frameworks like Spring MVC, and skills in HTML, CSS, and JavaScript.
- Database Management: Experience with relational databases such as MySQL, Oracle, or PostgreSQL, and SQL skills for database integration and manipulation.
- API Development: Ability to design and implement RESTful services and understanding of web services integration.
- Testing and Debugging: Skills in using testing frameworks like JUnit and Mockito for unit and integration testing to ensure code quality and reliability.
- Software Design Patterns: Understanding of patterns like Singleton, Factory, Strategy, and Observer, which are crucial for creating efficient and scalable software architectures.
- Version Control Systems: Proficiency with tools like Git for source code management, which is essential for collaborative development environments.
- Agile and Scrum Methodologies: Familiarity with Agile practices and experience working in Scrum teams to deliver projects iteratively and efficiently.
Need to Hire Java Developers?
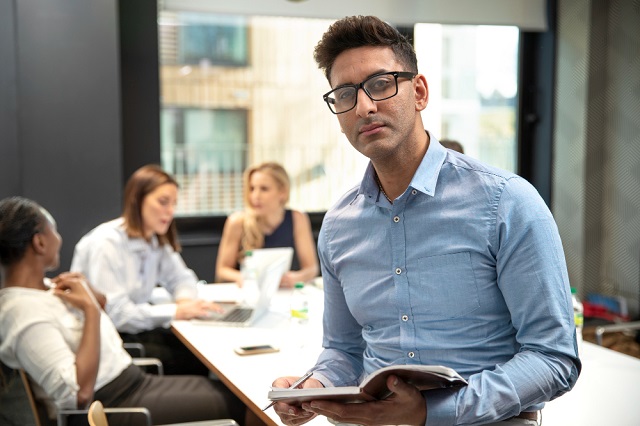
If you’re looking to enhance your team with skilled Java Developers, Tier2Tek Staffing can connect you with top-tier talent suited to your project needs. We specialize in finding expert developers who can bring value and innovation to your business.
- Sourcing Speed: Fast delivery of qualified Java Developers.
- Communication with Clients: Constant, clear communication to meet your exact needs.
- Quality Candidates: Only the most proficient Java Developers are presented.
- Innovative Sourcing Strategies: Advanced techniques to find the perfect match for skills and culture.